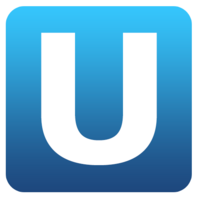
UniLib is the Universal Library for Rising World plugins. It has modular structure, which means that its functionality is separated into smaller subprojects: if you don't need the whole library functionality for your plugin you can just select modules that you need. Modules are supposed to be used as embedded libraries (packed inside plugin jar).
Links:
- Project License: MIT
- Project Sources
- Project Wiki
Releases:
- JitPack
- GitHub
Available Modules:
1. unilib-database-utils
- Database management module
- Create databases, tables and requests with builders
- Locate databases in world, plugin or custom directories
- Store different data types (including Vector3f and Vector3i)
- Update, remove and add new data into tables with builders
- Don't require SQL syntax knowledge
2. unilib-config-utils
- Config management module
- Create simple configs in .conf format (in plugin folder)
- Store primitives and custom classes
- Comment any entry with as many comments as needed
3. unilib-terrain-materials
- Terrain Material data storage module
- Contains all terrain materials as constants with IDs, names and average colors
- Have additional methods to get terrain by ID/name or get grass by length
Planned Modules:
- math module - some useful mathematical operations and fast trigonometric functions
- blueprint module - integrated version of BlueLib to work with RW blueprints
- noise module - module with different noise functions (perlin, simplex, voronoi)
- sdf module - module with SDF functions (mostly ported from BCLib with adaptations)
- worldgen module (when API will be updated) - to manipulate worldgen (probably will be not necessary)