Also ich habe mal etwas mit dem Prefab herum Gesspielt
Das Project ist noch im Versuchs Sdadium, also ein wenig Kaotisch 
VFX
mit einem Unterordner [1.] vfx_Spawn und dem [2.] VFX im Prefab, kann ich wunderbar die Steuerung nutzen.
lokalPrefabRauch.playVFX("vfx_Spawn");
lokalPrefabRauch.stopVFX("vfx_Spawn");
lokalPrefabRauch.reinitVFX("vfx_Spawn");
Animator
Der [2.] Animator läst sich ebenfalls wunderbar [3.] Steuern.
lokalPrefab.setAnimatorParameter("Neu_Mimic_gehen", "walk", true);
lokalPrefab.setAnimatorParameter("Neu_Mimic_gehen", "walkSpeed", 1.25f );
Shader
Selbst erstellte Shader sind ebenfalls gut zu Steuern.
Zuerst war ich ein wenig Verwirrt da es kein Boolean Parameter gibt, aber als ich mich getraut habe den mit dem Integer (0/1) zu benutzen hat es ebenfalls geklappt.
lokalPrefab.setMaterialParameter("Neu_Mimic_gehen/Cylinder.002", "_Albedo", new TextureAsset() );
lokalPrefab.setMaterialParameter("Neu_Mimic_gehen/Cylinder.002", "_hasFresnel", 1);
lokalPrefab.setMaterialParameter("Neu_Mimic_gehen/Cylinder.002", "_FresnelColor", new Vector4f() );
lokalPrefab.setMaterialParameter("Neu_Mimic_gehen/Cylinder.002", "_FresnelPower", 2.25f);
Animator 2
Ich kann auch den Shader Animieren, nur dazu muss der Animator auf den Richtigen Unterordner angepasst sein (Unity interner)
lokalPrefab.setAnimatorParameter("", "goOn", true);
red51 Jetzt habe ich nur das Problem das ich wenn der "Animator 2"[2.] Aktiv ist, kann ich nicht mehr "Shader"[2.] nutzen, warscheinlich überschreit die Animation die werte. Ich Habe versucht ihn mit der API zu Aktivieren oder Deaktivieren, leider ohne erfolg.
lokalPrefab.stopAnimatorPlayback("");
lokalPrefab.setComponentEnabled("", "_Animator", true); //auch schon mit "_animator"
wo ist hier mein Denkfehler oder ist es noch nicht Fertig Implementiert, wie benutze ich die Richtig?
Was ich auch noch gerne wüste, was mache ich mit invokeComponentMethod, klingt Spannend, aber konnte da, noch nix mit bewirken.
Was ich mir noch Dringend
wünsche ist das auslesen des Transform und eine Liste der enthaltenen Unterordner
also jeden Tranform im Prefab mit Namen
Ich möchte Quasie Spezielle Pivot Punkte Setzen, um z.B. bei einem Stuhl die Sitzfläche oder die Auflagefläche für die Hand an der Armlehne zu ermitteln, Modulbauweise Elemente einrasten Lassen.
Die ich dann in der API auslesen kann und damit andere Bauteile genauer zu Platzieren kann.
Oder gibt es für soetwas einen besseren Ansatz, als mit den Unterordnern? Eine Aksen/Tranform Liste im Stile einer MashMap/List?
Ich versuche ja gerade einen NPC zu erstellen, dafür nutze ich gerade ein Timer um die Bewegungen und Richtungen zu Koordienieren.
Dabei ist mir aufgefallen das ein Timer nicht das Optimale ist, besser wehre es wenn ich mit Events Arbeiten könnte (Angekommen,Kolliedirt mit, und weiteres), das würde die Performance verbessern.
Allerdings bräuchte man schon eien "kontinuirlichen Event" um z.B. die entfernung zum Spieler zu ermitteln, der müsste aber nicht auf jedem Tick hängen, vieleicht nur bei Spieler Bewegung, Change World, Load/Spawn, MoveToFinish
Wenn du schon ein wenig zu NPC's und API sagen könntest
wehre das Super.
In Trello sieht es gerade nicht danach aus das es geplant ist
Was mich aber schon eine weile Verrückt macht ist, der Schatten
Wie hast du das gemacht das die vom Licht abgewante Seite nicht im Schwarzen Schatten Versinkt?
Hier habe ich einfach mal ein Shader gebaut der die Basistexture Abdunkelt und als Emissiv raus gibt
das macht es erstmal
Ist das der Weg den du auch genommen hast?
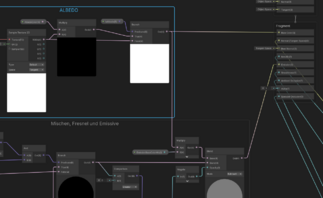
Wenn du für die UI noch die Liste und Dropdown freigeben könntest wehre das Wunderbar
Oder für die Nutzung von USS & UXML, noch einen kleinen Tip gibst, wie mann das geladen bekommt wenn beide StyleSheetAsset vorhanden sind